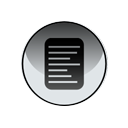
The PushRegistry maintains a list of inbound connections. An application can register the inbound connections with an entry in the JAD file or by dynamically calling the registerConnection method. If a notification arrives for a registered MIDlet when the application is not running, the AMS will start the MIDlet via the MIDlet.startApp() method.
/*Display the list of all inbound connections.*/
public void displayConnections() {
String[] allConnections = PushRegistry.listConnections(false);
StringBuffer sbuf = new StringBuffer();
if (allConnections != null && allConnections.length > 0) {
for (int i = 0; i < allConnections.length; i++ ) {
sbuf.append(allConnections[i]);
sbuf.append("\n");
}
}
String str = sbf.toString();
}
/*Register or Unregister an inbound connection*/
public void regConnection() {
try {
String url = "socket://";
String classname = "Midlet" //Midlet to be launched
if (register) {
PushRegistry.registerConnection(url, classname, "*");
} else {
PushRegistry.unregisterConnection(url);
}
} catch (IllegalArgumentException iae) {
} catch (IOException ioe) {
}
}
/*register an alarm*/
public void setAlarm() {
try {
Date d = new Date();
String classname = "Midlet"
long previousAlarmTime = PushRegistry.registerAlarm(
classname,d.getTime() + MILLISECONDS_TILL_ALARM);
} catch (Exception e) {
}
}
/*Connect to Url*/
public void connectUrl() {
try {
String[] connections = PushRegistry.listConnections(true);
if (connections != null && connections.length > 0) {
for (int i = 0; i < connections.length; i++ ) {
ServerSocketConnection serverConnection =
(ServerSocketConnection)Connector.open(connections[i]);
SocketConnection socketConnection =
(SocketConnection)serverConnection.acceptAndOpen();
socketConnection.close();
serverConnection.close();
}
}
} catch (Exception e) {
}
}
No comments:
Post a Comment
Place your comments here...