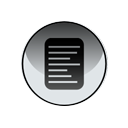
This function scales images using an optimized form of bresenham's formula. You can also add bilinear sampling and such to it but I figured since it's on a cell phone it won't matter much and will also cause it to be slower.
public static Image scaleImage( Image original, int newWidth, int newHeight) { int[] rawInput = new int[original.getHeight() * original.getWidth()]; original.getRGB(rawInput, 0, original.getWidth(), 0, 0, original.getWidth(), original.getHeight()); int[] rawOutput = new int[newWidth * newHeight]; // YD compensates for the x loop by substracting the width //back out. int YD = (original.getHeight() / newHeight) * original.getWidth() – original.getWidth(); int YR = original.getHeight() % newHeight; int XD = original .getWidth() / newWidth; int XR = original.getWidth() % newWidth; int outOffset = 0; int inOffset = 0; for (int y= newHeight, YE= 0, y > 0; y--) { for (int x= newWidth, XE= 0; x > 0; x--) { rawOutput[outOffset++] = rawInput[inOffset]; inOffset += XD; XE += XR; if (XE >= newWidth) { XE –= newWidth; inOffset++; } } inOffset += YD; YE += YR; if (YE >= newHeight) { YE –= newHeight; inOffset += original.getWidth(); } } return Image.createRGBImage(rawOutput, newWidth, newHeight, false); } |
boss will u share your email Id
ReplyDelete